MATLAB Onramp isn’t just another coding course; it’s your gateway to unlocking the power of MATLAB. This program’s designed for absolute beginners, offering a clear path to mastering essential concepts and techniques. Think of it as your friendly guide to the world of data analysis, visualization, and programming, all within the intuitive environment of MATLAB. Whether you’re an engineering student, a budding scientist, or just curious about data, Onramp provides the foundation you need to start building cool projects.
Table of Contents
From understanding basic variables and data types to tackling more advanced topics like loops and functions, Onramp systematically builds your skills. You’ll learn to import and export data, visualize results, and even troubleshoot those pesky errors that always seem to pop up. By the end, you’ll be equipped to tackle real-world problems and confidently explore the vast potential of MATLAB.
Introduction to MATLAB Onramp
MATLAB Onramp is a free online introductory course designed to get you up and running with MATLAB quickly and efficiently. It’s perfect for anyone who’s new to programming or just needs a fast track to using MATLAB for their work or studies. Think of it as your friendly, fast-paced introduction to a powerful tool.The target audience is incredibly broad.
Students across various disciplines (engineering, science, math, etc.) find it invaluable, as do researchers, data analysts, and anyone needing to analyze data or build models. No prior programming experience is required; the course is structured to bring you up to speed regardless of your background.
Key Features and Functionalities Covered
MATLAB Onramp covers the fundamental elements necessary to start using MATLAB effectively. This includes a solid introduction to the MATLAB environment, teaching you how to navigate the interface and utilize its core functionalities. You’ll learn about variables, data types, and basic operations. The curriculum also delves into essential programming concepts such as loops, conditional statements, and functions. Crucially, the course emphasizes practical application, teaching you how to create scripts, work with matrices (a fundamental data structure in MATLAB), and visualize data through plots and graphs.
Expect to work through numerous hands-on exercises designed to solidify your understanding. Specific topics often include creating and manipulating arrays, using built-in functions for mathematical operations and data analysis, and developing simple algorithms.
Benefits of Using MATLAB Onramp for Beginners
MATLAB Onramp offers several significant advantages for beginners. Firstly, its self-paced nature allows learners to progress at their own speed, revisiting concepts as needed. The interactive lessons and exercises provide immediate feedback, allowing for quick identification and correction of errors. This iterative learning process significantly improves understanding and retention. The course’s focus on practical application ensures that learners develop hands-on skills, applying what they learn immediately.
This contrasts with theoretical-heavy courses, where the practical application may be delayed or absent. Finally, the free and readily available nature of the program makes it accessible to a wider audience, removing financial barriers to entry. For instance, a student exploring potential career paths in engineering could use Onramp to determine if MATLAB is a good fit before investing in more extensive training.
Core Concepts Covered in MATLAB Onramp
MATLAB Onramp provides a foundational understanding of MATLAB’s core functionalities, equipping users with the essential tools for various applications. It covers the basic syntax, data structures, and operations crucial for building more complex programs. This section details the key concepts introduced.
Basic MATLAB Syntax and Commands
MATLAB’s syntax is relatively straightforward. Commands are typed directly into the command window, and the results are displayed immediately. For example, basic arithmetic operations are performed using standard symbols: `+`, `-`, `*`, `/`, and `^` for addition, subtraction, multiplication, division, and exponentiation respectively. More complex operations can be achieved using built-in functions like `sin()`, `cos()`, `sqrt()`, and many others.
These functions take numerical inputs and return corresponding outputs. For instance, `sqrt(25)` will return 5. Assigning values to variables is done using the `=` operator. For example, `x = 5;` assigns the value 5 to the variable `x`. Semicolons suppress output to the command window.
Variables, Data Types, and Operators
MATLAB supports various data types, including numbers (integers, floating-point), characters, strings, and logical values (true/false). Variables are used to store data. The data type of a variable is automatically determined based on the assigned value. For example, `x = 5` creates a numeric variable, while `name = ‘John’;` creates a string variable. MATLAB also offers a range of operators: arithmetic (as mentioned above), relational (`==`, `~=`, ` <`, `>`, `<=`, `>=`), logical (`&&`, `||`, `~`), and assignment (`=`). These operators are used to perform comparisons, logical operations, and assignments. The use of parentheses `()` to control the order of operations is essential for ensuring accurate calculations. For instance, `(2 + 3)
- 4` will be evaluated differently than `2 + 3
- 4`.
Matrices and Arrays
MATLAB excels in handling matrices and arrays. These are fundamental data structures in MATLAB. Arrays are ordered collections of elements, while matrices are two-dimensional arrays. Creating arrays is straightforward. For example, `a = [1 2 3 4];` creates a row vector, while `b = [1; 2; 3; 4];` creates a column vector.
Matrices are created using semicolons to separate rows. For example, `A = [1 2; 3 4];` creates a 2×2 matrix. MATLAB provides numerous built-in functions for manipulating matrices and arrays, such as calculating the transpose (`A.’`), finding the determinant (`det(A)`), and performing matrix multiplication (`AB`). These functions are extensively used in linear algebra and other scientific computations.
Control Flow
MATLAB supports standard control flow structures such as `if-else` statements and `for` and `while` loops. `if-else` statements allow conditional execution of code blocks. For example:
`if x > 0 disp(‘x is positive’);else disp(‘x is not positive’);end`
`for` loops iterate over a sequence of values, while `while` loops repeat as long as a condition is true. These structures are crucial for creating programs that perform repetitive tasks or make decisions based on conditions. For example, a `for` loop can be used to calculate the sum of numbers in an array, and a `while` loop can be used to simulate a process that continues until a certain threshold is reached.
Functions
Functions are reusable blocks of code that perform specific tasks. They enhance code organization, readability, and reusability. A simple function can be defined as follows:
`function y = myFunction(x) y = x^2;end`
This function takes a single input `x` and returns its square. Functions can take multiple inputs and return multiple outputs. They are crucial for modularizing code and making it easier to maintain and extend. Well-structured functions significantly improve the efficiency and clarity of larger MATLAB programs.
Working with Data in MATLAB Onramp
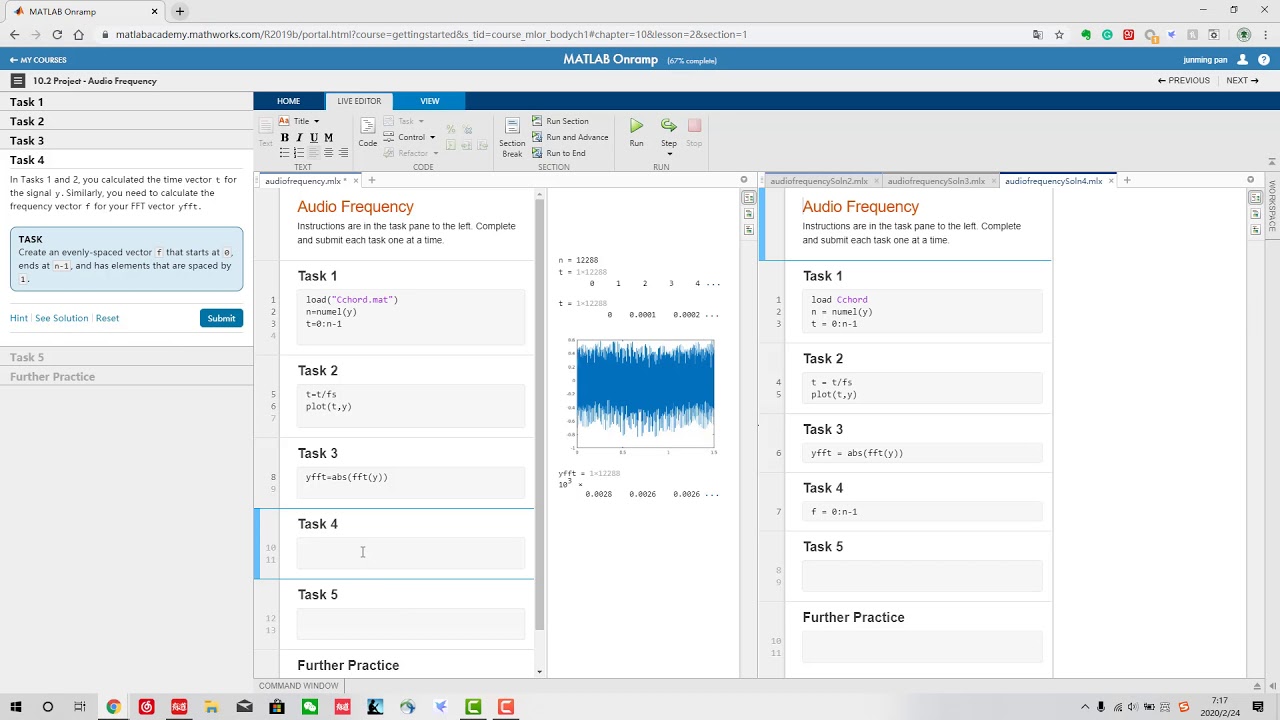
MATLAB’s power lies in its ability to handle and manipulate data efficiently. This section explores how the Onramp course introduces you to importing, exporting, manipulating, and visualizing data, laying the groundwork for more advanced data analysis techniques. We’ll cover essential functions and methods to make your data wrangling smoother and more intuitive.
Data import and export are fundamental steps in any data analysis workflow. MATLAB provides a wide array of functions to seamlessly interact with various data formats. From spreadsheets and text files to specialized scientific formats, MATLAB simplifies the process of getting your data into and out of the workspace. Data manipulation techniques, such as filtering, sorting, and transforming data, are equally crucial for preparing your data for analysis and visualization.
Finally, visualizing data through plots and charts is essential for gaining insights and communicating findings effectively.
Importing and Exporting Data
MATLAB offers a variety of ways to import and export data. The `importdata` function is a versatile tool for importing data from various file types, including text files, spreadsheets, and images. For specific file formats like MAT-files (MATLAB’s native format), dedicated functions like `load` and `save` offer optimized performance. Exporting data is equally straightforward, with functions like `csvwrite` and `xlswrite` allowing you to easily save data to comma-separated value files and Excel spreadsheets, respectively.
For example, `load(‘mydata.mat’)` loads data from a MAT-file named ‘mydata.mat’, while `csvwrite(‘output.csv’, myData)` writes data stored in the variable `myData` to a CSV file named ‘output.csv’. The choice of function depends on the specific file format and the efficiency requirements of your workflow.
Data Manipulation Techniques
Once data is imported, manipulating it is often necessary. MATLAB provides a rich set of functions for this purpose. For example, `sort` sorts data in ascending or descending order; `find` locates elements that satisfy a specific condition; and logical indexing allows for selective extraction of data based on criteria. Matrix operations, such as element-wise multiplication (`.*`) and matrix multiplication (`*`), are fundamental for manipulating numerical data.
Consider a dataset stored in a matrix; you might use `find(matrix > 10)` to find indices of elements greater than 10, or `matrix(find(matrix > 10)) = 0` to set those elements to zero. These functions enable efficient data cleaning, transformation, and feature engineering.
Data Visualization
Visualizing data is key to understanding patterns and trends. MATLAB provides a wide array of plotting functions. `plot` creates 2D line plots, `scatter` generates scatter plots, and `bar` creates bar charts. More advanced plots, like 3D surface plots (`surf`) and contour plots (`contour`), are also readily available. Each function offers customization options for labels, titles, colors, and legends, enabling the creation of informative and visually appealing graphics.
For instance, `plot(x,y)` creates a simple line plot, while `scatter(x,y,[],’red’)` creates a scatter plot with red markers. Proper visualization is crucial for communicating insights derived from data analysis.
So, you’re crushing the Matlab Onramp, right? Learning all those matrix manipulations is killer, but sometimes you need to move beyond basic simulations. That’s where software like abaqus comes in handy for more complex finite element analysis. Then, once you’ve got a handle on the FEA results, you can import them back into Matlab Onramp for further processing and visualization – it’s a powerful workflow!
Table of Data Manipulation Techniques
The following table summarizes some common data manipulation techniques and their corresponding MATLAB functions:
Technique | MATLAB Function(s) | Description | Example |
---|---|---|---|
Sorting | sort |
Sorts data in ascending or descending order. | sortedData = sort(data); |
Filtering | find , logical indexing |
Selects data based on specified criteria. | filteredData = data(data > 10); |
Transformation | log , sqrt , etc. |
Applies mathematical functions to data. | transformedData = log(data); |
Reshaping | reshape |
Changes the dimensions of a matrix or array. | reshapedData = reshape(data, [5, 10]); |
Programming Fundamentals in MATLAB Onramp
MATLAB’s power extends beyond simple calculations; it’s a full-fledged programming language. This section dives into the fundamental programming constructs that allow you to create sophisticated and efficient MATLAB programs. We’ll cover control flow, which dictates the order of execution, and functions, which encapsulate reusable code blocks.
Control Flow Structures
Control flow structures determine the order in which your MATLAB code executes. Instead of running line by line, you can create programs that make decisions and repeat actions based on conditions or iterations. This significantly increases the complexity and utility of your programs. Two key structures are conditional statements (like `if`, `elseif`, `else`) and loops (like `for` and `while`).
Conditional Statements
Conditional statements allow your code to execute different blocks of code based on whether a condition is true or false. The basic structure is: if condition % Code to execute if condition is trueelseif anotherCondition % Code to execute if anotherCondition is trueelse % Code to execute if no conditions are trueend
For example, consider a program that determines if a number is positive, negative, or zero: num = -5;if num > 0 disp('The number is positive.');elseif num < 0
disp('The number is negative.');
else
disp('The number is zero.');
end
This code will display "The number is negative." because the variable `num` holds the value -5.
Loops
Loops allow you to repeatedly execute a block of code. `for` loops iterate a specific number of times, while `while` loops continue as long as a condition is true.A `for` loop example: for i = 1:5 disp(i);end
This will display the numbers 1 through 5, each on a new line.A `while` loop example: i = 1;while i <= 5
disp(i);
i = i + 1;
end
This also displays the numbers 1 through 5, but the loop continues until the condition `i <= 5` becomes false. It's crucial to ensure your `while` loop condition eventually becomes false to avoid infinite loops.
Creating and Using Functions in MATLAB
Functions are self-contained blocks of code that perform a specific task.
They improve code organization, reusability, and readability. A function takes input arguments, performs calculations or operations, and returns output values.The basic structure of a MATLAB function is: function [outputArgs] = myFunction(inputArgs) % Code to perform the function's taskend
For example, a function to calculate the area of a rectangle: function area = rectangleArea(length, width) area = length - width;end
This function takes the length and width as input and returns the calculated area. You would call this function like this: myArea = rectangleArea(5, 10); % myArea will be 50
Simple MATLAB Program Demonstrating Loops and Conditional Statements
Let's create a program that simulates a simple dice roll game. The program will roll a six-sided die (generating a random number between 1 and 6) multiple times and report whether each roll is even or odd. numRolls = 10; % Number of times to roll the diefor i = 1:numRolls roll = randi([1, 6]); % Generate a random integer between 1 and 6 disp(['Roll ', num2str(i), ': ', num2str(roll)]); % Display the roll number and the result if mod(roll, 2) == 0 disp('Even'); else disp('Odd'); endend
This program uses a `for` loop to simulate multiple dice rolls. Inside the loop, a random number is generated, displayed, and then a conditional statement checks if the number is even or odd, displaying the appropriate message.
This demonstrates a practical application of both loops and conditional statements in MATLAB.
MATLAB Onramp and its Applications
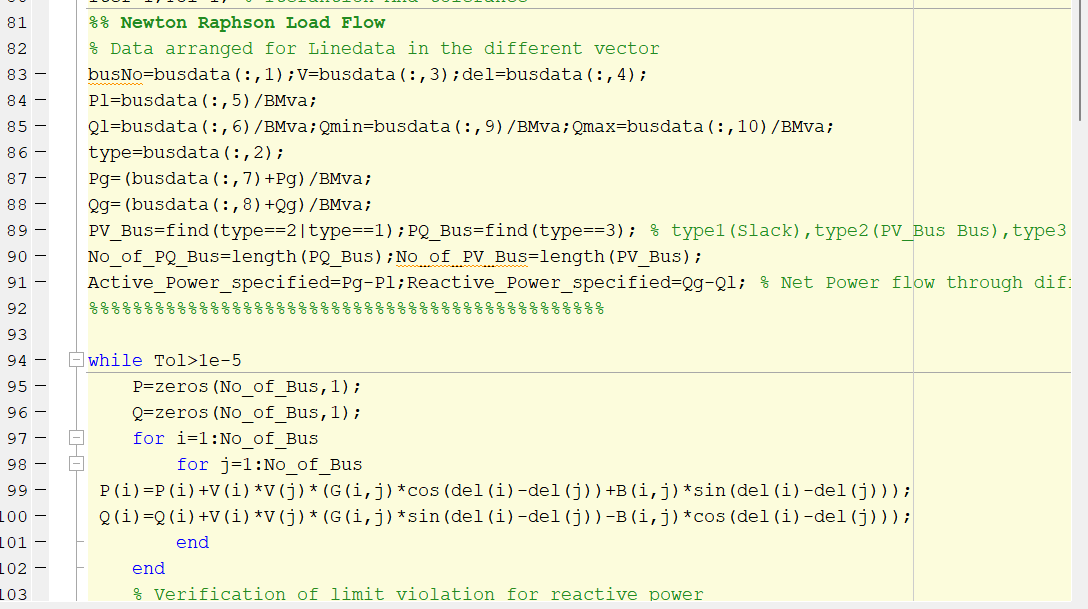
MATLAB's power lies not just in its ease of use but in its incredibly broad applicability across diverse fields. It's a tool used by researchers, engineers, and financial analysts alike to solve complex problems and visualize data in intuitive ways. Its versatility stems from its extensive toolboxes, which provide specialized functions for specific domains.MATLAB's ability to handle large datasets, perform complex calculations, and create compelling visualizations makes it an invaluable asset in many industries.
This section will explore some key application areas and illustrate how MATLAB tackles real-world challenges.
Engineering Applications
MATLAB is ubiquitous in engineering. Its numerical computation capabilities are ideal for simulating systems and analyzing data from experiments. For example, in mechanical engineering, finite element analysis (FEA) simulations are commonly performed using MATLAB toolboxes to model stress and strain in complex structures, like bridges or aircraft components. Electrical engineers use MATLAB for circuit design and analysis, simulating the behavior of circuits before physical prototyping.
Control systems engineers leverage MATLAB's control system toolbox to design and analyze feedback control systems, crucial for applications like robotics and autonomous vehicles.
- Mechanical Engineering: FEA simulations for structural analysis, predicting component failure, optimizing designs.
- Electrical Engineering: Circuit simulation and design, signal processing, digital signal processing (DSP) algorithm development.
- Aerospace Engineering: Flight dynamics simulation, aerodynamic modeling, control system design for aircraft and spacecraft.
Scientific Applications
MATLAB's strength in data analysis and visualization makes it a powerful tool for scientific research. Researchers across various disciplines use MATLAB to process experimental data, develop and test models, and create publication-quality figures. For instance, in biology, MATLAB is used for image analysis of microscopy data, helping researchers identify cells or track biological processes. In physics, MATLAB can simulate physical phenomena, such as fluid flow or particle interactions.
- Biomedical Engineering: Image analysis of medical images (MRI, CT scans), signal processing of physiological data (ECG, EEG).
- Physics: Simulation of physical systems, data analysis from experiments, development of scientific models.
- Chemistry: Data analysis from spectroscopic techniques, modeling chemical reactions, predicting molecular properties.
Financial Applications
The financial industry uses MATLAB for quantitative analysis, risk management, and algorithmic trading. MATLAB's ability to handle large financial datasets and perform complex statistical calculations makes it well-suited for tasks such as portfolio optimization, risk assessment, and derivative pricing. For example, financial analysts might use MATLAB to build models that predict stock prices or assess the risk associated with a particular investment strategy.
- Quantitative Finance: Portfolio optimization, risk management, derivative pricing, algorithmic trading.
- Financial Modeling: Building predictive models for stock prices, interest rates, or other financial instruments.
- Risk Management: Assessing and mitigating financial risks, such as credit risk or market risk.
Troubleshooting Common MATLAB Errors in Onramp
So, you're diving into MATLAB Onramp and hitting a few snags? Don't worry, it happens to everyone! MATLAB, like any powerful programming language, can throw some curveballs, especially when you're just starting out. This section will cover some common errors beginners encounter and provide strategies to help you navigate them. The key is understanding how to read and interpret error messages—they're your best friend in debugging.
Understanding MATLAB Error Messages
MATLAB error messages might seem intimidating at first, but they're actually incredibly helpful. They typically provide a concise description of the problem, the line of code where the error occurred, and sometimes even a suggestion for fixing it. For example, an "Index exceeds matrix dimensions" error means you're trying to access an element in an array that doesn't exist.
Pay close attention to the line number—that's your starting point for debugging. Don't just skim the message; read it carefully! Often, the solution is staring you right in the face.
Common Errors and Their Solutions
Let's look at a few common MATLAB pitfalls for Onramp users.
Error Type | Description | Solution | Example |
---|---|---|---|
Undefined function or variable | MATLAB can't find a function or variable you're using. This often happens due to typos, incorrect capitalization, or forgetting to define a variable before using it. | Double-check your spelling and capitalization. Ensure the variable is defined before being used. If it's a function, make sure it's in your MATLAB path or that you've correctly called it. | >> myVariable = 10; >> MyVariable + 5; %Error: Undefined function or variable 'MyVariable'. The solution is to maintain consistent capitalization (myVariable ). |
Index exceeds matrix dimensions | You're trying to access an element in an array that's outside its bounds (e.g., trying to access the 10th element of a 5-element array). | Carefully check the size of your arrays using the size() function. Make sure your loop indices or array accesses are within the valid range. |
>> myArray = [1 2 3 4 5]; >> myArray(6) %Error: Index exceeds matrix dimensions. The solution is to ensure that the index is within the bounds of the array (1 to 5). |
Matrix dimensions must agree | This error occurs when you try to perform an operation (like matrix multiplication) on matrices with incompatible dimensions. | Verify that the dimensions of your matrices are compatible with the operation you're performing. Use the size() function to check dimensions. |
>> A = [1 2; 3 4]; >> B = [5 6 7]; >> A
The solution is to ensure that the number of columns in A matches the number of rows in B for matrix multiplication. |
Missing semicolon | Forgetting a semicolon at the end of a line of code can lead to unexpected output or errors, especially when dealing with large datasets or loops. | Always use semicolons to suppress output, except when you explicitly want to see the results of a command. | >> for i = 1:1000 >> disp(i) >> end % Produces 1000 lines of output. Adding semicolons after disp(i) suppresses this. |
Using the Debugger
MATLAB's built-in debugger is a powerful tool. It allows you to step through your code line by line, inspect variable values, and identify the exact point where errors occur.
Learning to use the debugger is an invaluable skill for any MATLAB programmer. It's like having a magnifying glass for your code! You can set breakpoints, step over or into functions, and watch how variables change as your code executes. This is particularly useful for tracking down subtle errors in more complex programs.
Preventing Errors: Good Coding Practices, Matlab onramp
Writing clean, well-commented code is key to preventing errors. Use meaningful variable names, break down complex tasks into smaller, more manageable functions, and always add comments to explain what your code is doing. This makes your code easier to read, understand, and debug. Think of it as writing for your future self (and anyone else who might look at your code).
A little extra time spent on clear coding saves a lot of debugging time later.
Advanced Topics Introduced in MATLAB Onramp
MATLAB Onramp doesn't just cover the basics; it also gives you a taste of some more advanced capabilities that can significantly expand your problem-solving toolkit. While it won't make you an expert, it provides enough exposure to pique your interest and point you towards further learning. These advanced features are often used in specialized fields and represent powerful tools for tackling complex problems.The advanced topics introduced often depend on the specific Onramp curriculum, but commonly include elements of symbolic mathematics and image processing.
These topics are introduced through concise examples and exercises, providing a foundational understanding of their potential. This allows users to appreciate the breadth of MATLAB's capabilities and to identify areas for further exploration based on their individual interests and research goals.
Symbolic Math in MATLAB Onramp
MATLAB's Symbolic Math Toolbox allows you to work with mathematical expressions symbolically, rather than numerically. This means you can manipulate equations, solve for variables, and perform calculus operations without relying on numerical approximations. For instance, you can find the derivative of a complex function, solve systems of equations analytically, or even perform symbolic integration. Onramp likely introduces basic symbolic operations like defining symbolic variables, performing differentiation and integration, and solving simple algebraic equations.
This foundation allows users to explore more complex mathematical manipulations in their own time. The ability to work symbolically can be incredibly useful in areas like control systems design, theoretical physics, and engineering analysis where exact solutions are often needed. For example, deriving a transfer function for a control system symbolically provides more insight than a numerical approximation.
Image Processing in MATLAB Onramp
MATLAB's Image Processing Toolbox provides a comprehensive set of functions for manipulating and analyzing images. Onramp likely provides a brief introduction to fundamental image processing operations, such as image reading, writing, display, basic filtering (like smoothing and sharpening), and perhaps some color space transformations. These operations are often visualized, giving the user a tangible understanding of how they impact the image data.
The relevance of image processing is vast, spanning fields like medical imaging (analyzing X-rays or MRIs), computer vision (object recognition), remote sensing (analyzing satellite imagery), and many more. A simple example from Onramp might involve applying a Gaussian filter to reduce noise in an image, demonstrating the practical application of image processing techniques.
Resources for Continued Learning
After completing MATLAB Onramp, several resources are available to deepen your understanding and explore more advanced topics. MATLAB's extensive documentation is a fantastic starting point, offering detailed explanations of functions, toolboxes, and best practices. The MathWorks website also provides numerous tutorials, examples, and online courses that cover various aspects of MATLAB, from beginner to advanced levels. Furthermore, there are countless online communities and forums where you can connect with other MATLAB users, ask questions, and share your knowledge.
Finally, many universities offer specialized courses in MATLAB and its applications, providing structured learning paths for those wishing to master specific toolboxes or applications.
Comparison of MATLAB Onramp with Other Learning Resources
MATLAB Onramp is a great introductory course, but it's not the only game in town when it comes to learning MATLAB. Several other resources exist, each with its own strengths and weaknesses. Choosing the right one depends on your learning style, budget, and specific goals. This section compares Onramp to some popular alternatives, highlighting key differences to help you make an informed decision.
Many resources cater to different learning styles and experience levels. Some are free, while others require a subscription or purchase. Content quality and user experience also vary widely.
MATLAB Documentation
The official MATLAB documentation is a comprehensive resource, providing detailed explanations of functions, toolboxes, and functionalities. It's free to access if you have MATLAB installed, making it a valuable supplement to any learning course. However, its sheer size and technical depth can be overwhelming for beginners. Onramp, in contrast, provides a more structured and guided learning path.
Online Courses (Coursera, edX, Udemy)
Platforms like Coursera, edX, and Udemy offer numerous MATLAB courses, ranging from beginner to advanced levels. These courses often include video lectures, assignments, and quizzes, providing a more interactive learning experience than simply reading documentation. However, the quality of these courses can vary significantly, and some may require a fee. Onramp's advantage lies in its specifically designed, focused approach to introductory concepts.
It's concise and directly addresses the fundamentals. Some online courses might cover broader topics, potentially diluting the focus on the core MATLAB skills.
MATLAB Books
Several textbooks are dedicated to teaching MATLAB. These books often offer a more in-depth treatment of specific topics than online courses or Onramp. They're great for reference and in-depth understanding, but can be less engaging than interactive learning platforms. They also represent a significant upfront cost. Onramp's online, interactive nature makes it a more accessible and engaging option for many learners.
Comparison Table
The following table summarizes the key differences between MATLAB Onramp and other learning resources. Note that the cost for online courses can vary widely depending on the specific course and platform.
Resource | Cost | Content Depth | User Experience |
---|---|---|---|
MATLAB Onramp | Free | Introductory | Interactive, guided |
MATLAB Documentation | Free (with MATLAB) | Comprehensive, detailed | Can be overwhelming for beginners |
Online Courses (e.g., Coursera, edX, Udemy) | Varies (Free to Paid) | Varies (Introductory to Advanced) | Interactive, varies in quality |
MATLAB Textbooks | Paid | In-depth, comprehensive | Can be less engaging than interactive resources |
Illustrative Examples of MATLAB Onramp Projects
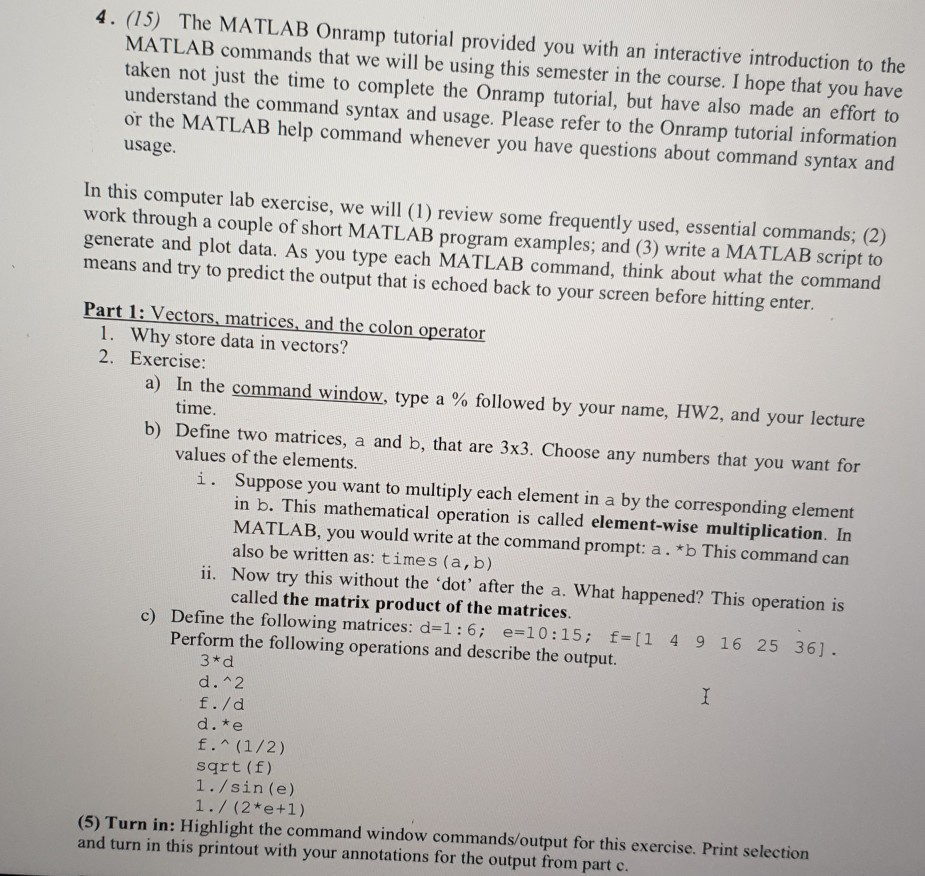
MATLAB Onramp provides a solid foundation for tackling a range of projects. By combining the core concepts – from basic operations to data visualization and programming – you can build surprisingly sophisticated applications. Let's look at a couple of examples to illustrate this.
Completing a simple MATLAB project often involves breaking down a problem into smaller, manageable steps. For example, imagine you need to calculate the area of a circle given its radius. You would first define the radius as a variable, then apply the formula (Ï€
- radius 2) using MATLAB's built-in functions and operators. Finally, you'd display the result.
This simple project demonstrates the use of variables, mathematical operations, and output functions, all fundamental aspects covered in Onramp.
A Data Analysis and Visualization Project: Analyzing Student Grades
This project analyzes a dataset of student grades across multiple subjects and visualizes the results. We'll assume the data is already imported into a MATLAB matrix, where each row represents a student and each column represents a different subject.
The first step involves calculating descriptive statistics for each subject, such as the mean, median, and standard deviation. MATLAB's built-in statistical functions make this straightforward. Next, we create visualizations to represent the data. A histogram could show the distribution of grades in each subject, while a box plot could compare the grade distributions across different subjects. Scatter plots could be used to explore correlations between pairs of subjects.
The choice of visualization depends on the specific insights we want to extract.
For instance, to create a histogram of grades in a specific subject (let's say, Math), you would use the histogram
function, providing the relevant column from the data matrix as input. To create a box plot comparing grades across all subjects, you'd use the boxplot
function with the entire data matrix. These visualizations allow for a quick and easy understanding of the overall performance and variations in student grades across subjects.
Finally, we might calculate the overall average grade for each student and identify students who performed exceptionally well or poorly. This involves matrix operations and conditional statements, allowing for a more in-depth analysis. The entire process, from data import and manipulation to visualization and analysis, effectively showcases the practical application of skills acquired during Onramp.
This project demonstrates the power of MATLAB for data analysis. By combining data manipulation, statistical analysis, and visualization techniques learned in Onramp, we can effectively analyze and understand complex datasets, revealing patterns and insights that would be difficult to discern manually. The use of MATLAB's built-in functions streamlines the process, allowing for efficient and accurate analysis.
Final Conclusion: Matlab Onramp
MATLAB Onramp is more than just a course; it's a launchpad. It equips you with the fundamental knowledge and practical skills to confidently navigate the world of MATLAB. You'll not only learn the basics but also gain the problem-solving skills necessary to tackle complex challenges. So dive in, explore, and discover the endless possibilities that await you in the world of data analysis and programming.
This is just the beginning of your coding adventure!
Essential FAQs
Is prior programming experience required for MATLAB Onramp?
Nope! Onramp is designed for beginners with no prior programming experience. They start from the very basics.
How long does it take to complete MATLAB Onramp?
The completion time varies depending on your pace and commitment, but it's structured to be manageable for busy schedules.
What kind of projects can I do after completing Onramp?
You'll be ready to tackle a wide range of projects, from simple data analysis to creating simulations and visualizations. The possibilities are pretty much limitless!
Are there any certifications or credentials offered upon completion?
Check the official MATLAB Onramp program details for information on any certifications or credentials they offer. This might vary.
What if I get stuck? Is there support available?
Most programs offer forums, online help, or even instructor support to help you troubleshoot issues and get unstuck. Check their support options.